Gameboy Advance Development
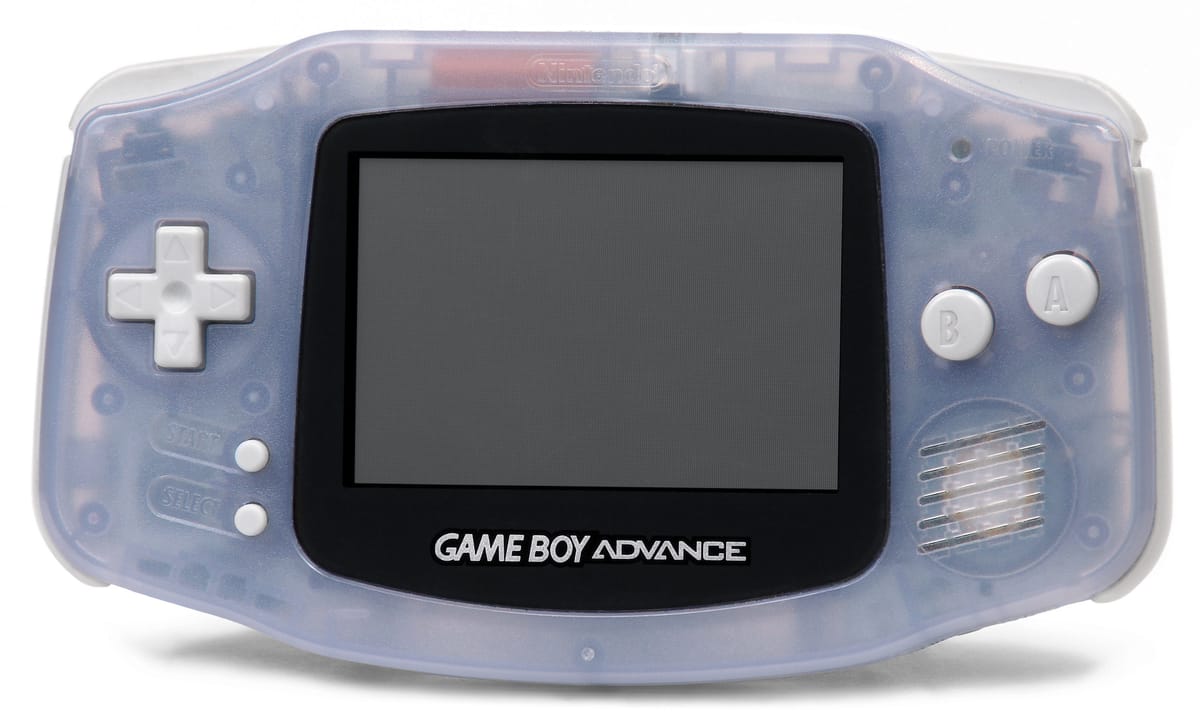
Students sometime ask me what is the best way to get into the game development business? Well, if you want to become a developper, you can help yourself a lot by getting some development experience with consoles. The easier console to learn happens to be the Gameboy Advance (GBA) and there is a ridiculous amount of information available for it. Here are some of my favorite links.
Note that GBA programming is not for the faint of heart. Although it is the easiest console, some serious C programming is required. Be warned.
A good place to start, gbadev has lots of links to tools and compilers you can use with the GBA
When working with the GBA, you are going to do some serious low-level programming. You need to understand the hardware. These are the two best resources out there.
Don't forget, Google is your best friend. Best of luck :-)
Photo by Evan Amos